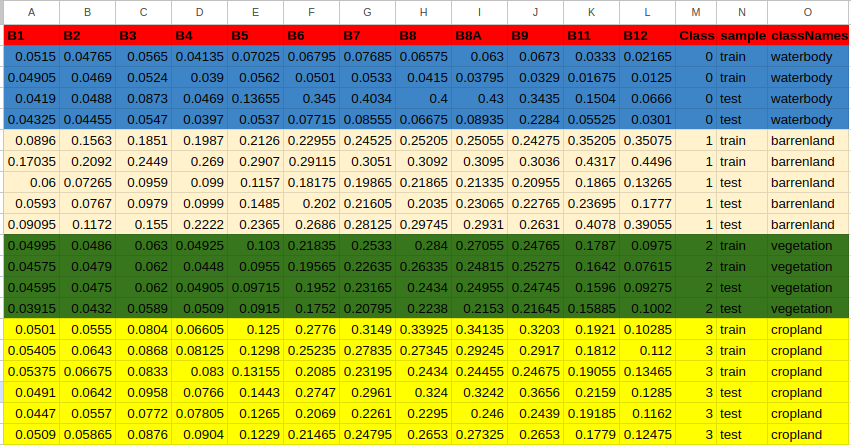
How to Export Training Samples with Band Values from Google Earth Engine
This blog post walks through a practical example of using Sentinel-2 data in Google Earth Engine (GEE) to create a training dataset for machine learning purposes. We’ll explore how to prepare your data, split it into training and testing sets, and export it for further analysis. we'll use Sentinel-2 imagery from Google Earth Engine to classify different land cover types. Our goal is to prepare a dataset that includes training and testing samples with specific band values. Here’s how to go about it:
- Load and Prepare Sentinel-2 Data
- Create and Merge Training Samples
- Split Samples into Training and Testing Sets
- Extract Values from the Sentinel-2 Image
- Export the Dataset
1. Load and Prepare Sentinel-2 Data
First, we need to load the Sentinel-2 imagery and preprocess it. We’ll filter the imagery based on date and cloud cover, select relevant bands, and apply necessary corrections.
// Visualization parameters for bands
var visparam = {bands: ['B4', 'B3', 'B2'], min: 338.14, max: 1544.86};
// Load Sentinel-2 image collection
var sentbhanda_2024 = ee.ImageCollection("COPERNICUS/S2_SR_HARMONIZED")
.filterDate('2023-12-01','2024-05-31')
.filterMetadata('CLOUDY_PIXEL_PERCENTAGE', 'less_than', 1)
.select(['B1', 'B2', 'B3', 'B4', 'B5', 'B6', 'B7', 'B8', 'B8A', 'B9', 'B11', 'B12', 'AOT', 'WVP'])
.median()
.clip(studyarea).multiply(0.0001);
// Print the resulting image collection
print(sentbhanda_2024, 'Image collection');
// Add the image to the map for visualization
Map.addLayer(sentbhanda_2024, visparam, 'sentbhanda_2024');
2. Create and Merge Training Samples
Training samples are essential for supervised machine learning. We define the classes of interest (waterbody, barrenland, vegetation, cropland) and create sample datasets for each class.
// Define class names and colors
var Class = [0, 1, 2, 3];
var className = ['waterbody', 'barrenland', 'vegetation', 'cropland'];
var columns = ['B1', 'B2', 'B3', 'B4', 'B5', 'B6', 'B7', 'B8', 'B8A', 'B9', 'B11', 'B12', 'Class', 'sample', 'classNames'];
var features = ['B1', 'B2', 'B3', 'B4', 'B5', 'B6', 'B7', 'B8', 'B8A', 'B9', 'B11', 'B12'];
// Merge training samples from different classes
var samples = waterbody.merge(barrenland).merge(vegetation).merge(cropland)
.map(function(feat){ return feat; });
print(samples);
3. Split Samples into Training and Testing Sets
Next, we split the samples into training and testing datasets. This step ensures that we can evaluate our model's performance on unseen data.
// Split sample to train and test per class
samples = ee.FeatureCollection(Class.map(function(value){
var features = samples.filter(ee.Filter.eq('Class', value)).randomColumn();
var train = features.filter(ee.Filter.lte('random', 0.8)).map(function(feat){
return feat.set('sample', 'train').set('classNames', className[value]);
});
var test = features.filter(ee.Filter.gt('random', 0.8)).map(function(feat){
return feat.set('sample', 'test').set('classNames', className[value]);
});
return train.merge(test);
})).flatten();
print('Samples after split:', samples);
4. Extract Values from the Sentinel-2 Image
With our samples prepared, we extract the pixel values from the Sentinel-2 imagery. These values will be used to train and test our machine learning model.
// Extract pixel values from the Sentinel-2 image
var extract = sentbhanda_2024.sampleRegions({
collection: samples,
scale: 10,
properties: ['sample', 'Class', 'classNames']
});
print('Extract:', extract);
5. Export the Dataset
Finally, we export the dataset as a CSV file to Google Drive, which can be used for training machine learning models.
// Export the dataset to Google Drive
Export.table.toDrive({
collection: extract,
fileFormat: 'CSV',
selectors: columns,
description: 'LULC_Points',
folder: 'GEE_MEDIAN',
});
Conclusion
In this blog post, we demonstrated how to prepare Sentinel-2 imagery for land cover classification using Google Earth Engine. By following these steps, you can create a robust training dataset for your machine learning models, enabling you to classify various land cover types accurately.
Stay tuned for more insights on how to leverage remote sensing data for advanced machine learning applications. Happy coding!
0% Positive Review (0 Comments)